A guide on IPFS file upload using Moralis Server as well as Cloud Functions
If you have no account on Moralis yet, get a free account as follows:
Go to Moralis Login
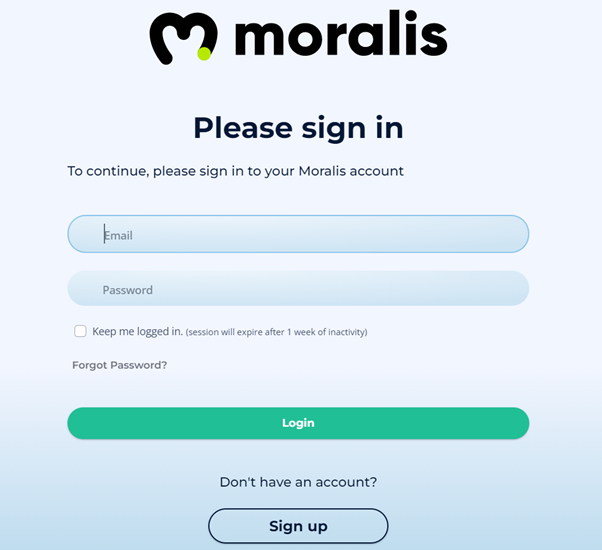
Create a new server

Select the chains to work with
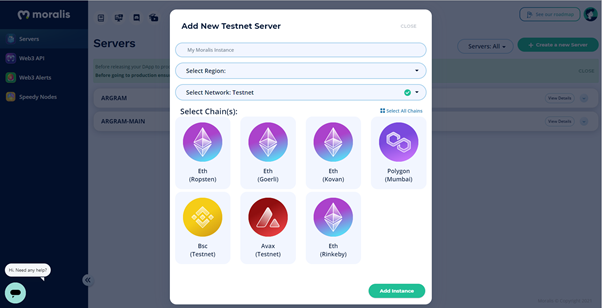
Copy Server Details(Server URL, Application ID and Master Key)
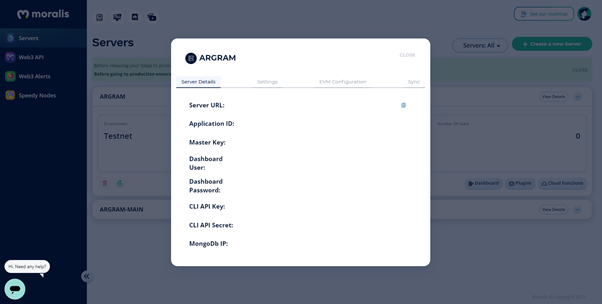
Start Moralis Server
require(“dotenv”).config(); const express = require(“express”); const Moralis = require(“moralis/node”); const app = express(); const { PORT, MORALIS_SERVER_URL, MORALIS_APP_ID, MORALIS_MASTER_KEY, } = process.env; const port = PORT || 8000; const upload = multer({ dest: __dirname + “/public/uploads/” }); app.use(express.urlencoded({ extended: true })); app.use(express.static(“public”)); app.listen(port, async () => { await Moralis.start({ serverUrl: MORALIS_SERVER_URL, appId: MORALIS_APP_ID, masterKey: MORALIS_MASTER_KEY, }); });
.env file should be like below:
MORALIS_SERVER_URL = `moralis server url`
MORALIS_APP_ID = `moralis application Id`
MORALIS_MASTER_KEY = `moralis master key`
Moralis provides two ways of approach to upload files to IPFS:
- Using saveIPFS() method of Moralis server
- Using Cloud Functions
1. Using saveIPFS() method of Moralis server
File Upload
const uploadFile = async (req, res, next) => { const { originalname: name, mimetype } = req.file; fs.readFile(req.file.path, async (err, buffer) => { const data = Array.from(Buffer.from(buffer, “binary”)); const file = new Moralis.File(name, data, mimetype); await file.saveIPFS({ useMasterKey: true }); return file.ipfs(); }); } ---------------------------------You will get a response of ipfs url like below: https://ipfs.moralis.io:2053/ipfs/QmdPcF1r86v28PeJMxdxHbKQA17qBhW3W5s6DHGvxukdPv
Json Upload
const uploadJson = async (req, res, next) => { const jsonMetadata = { name: "Female Bust", description: "Female Bust 3D model", image: "https://ipfs.moralis.io:2053/ipfs/QmdPcF1r86v28PeJMxdxHbKQA17qBhW3W5s6DHGvxukdPv"} const toBtoa = Buffer.from(JSON.stringify(jsonMetadata)).toString("base64"); const file = new Moralis.File("FemaleBust.json", { base64: toBtoa }); await file.saveIPFS({ useMasterKey: true }); return file.ipfs(); } ---------------------------------You will get a response of ipfs url like below: https://ipfs.moralis.io:2053/ipfs/QmdPcF1r86v28PeJMxdxHbKQA17qBhW3W5s6DHGvxukdPv
2. Using Cloud Functions
Format of a normal cloud function should be as below:
Function Definition
-----------------------------Moralis.Cloud.define(function_name, async (request) => {});You can access the params like request.params.[key]
Function call
------------------------------
const params = {"key": "value"};await Moralis.Cloud.run(function_name, params);
Create a folder /cloud and a file cloud.js inside it.
Write your cloud functions on that file.
Moralis.Cloud.define(“ipfsbinary”, async (request) => { const result = await Moralis.Cloud.define(“ipfsbinary”, async (request) => { const result = await Moralis.Cloud.toIpfs({ sourceType: “base64Binary”, source: request.params.image, }); return result; }); Moralis.Cloud.define(“ipfsjson”, async (request) => { const result = await Moralis.Cloud.toIpfs({ sourceType: “object”, source: request.params.metadata, }); return result; });
Now we can call the cloud functions from our file.
File Upload
const uploadFile = async (req, res, next) => { const uploadFile = async (req, res, next) => { fs.readFile(req.file.path, async (err, buffer) => { const image = buffer.toString(“base64”); const ipfsImagePath = await Moralis.Cloud.run(“ipfsbinary”, { image }); return ipfsImagePath; }); } ------------------------------------------ You will get a response like below: { path: "https://ipfs.moralis.io:2053/ipfs/QmdPcF1r86v28PeJMxdxHbKQA17qBhW3W5s6DHGvxukdPv" }
Json Upload
const uploadJson = async (req, res, next) => { const metadata = { name: "Female Bust", description: "Female Bust 3D model", image: "https://ipfs.moralis.io:2053/ipfs/QmdPcF1r86v28PeJMxdxHbKQA17qBhW3W5s6DHGvxukdPv" }; const ipfsJsonPath = await Moralis.Cloud.run(“ipfsjson”, { metadata}); return ipfsJsonPath; } ------------------------------------------ You will get a response like below: { path: "https://ipfs.moralis.io:2053/ipfs/QmdPcF1r86v28PeJMxdxHbKQA17qBhW3W5s6DHGvxukdPv" }