A guide on how to verify smart contract on mumbai polygonscan using hardhat configuration
This article deals with how we can verify the smart contract on mumbai polygonscan using hardhat.
Let’s dive into the code!
Step 1: Create an account in polygonscan.com
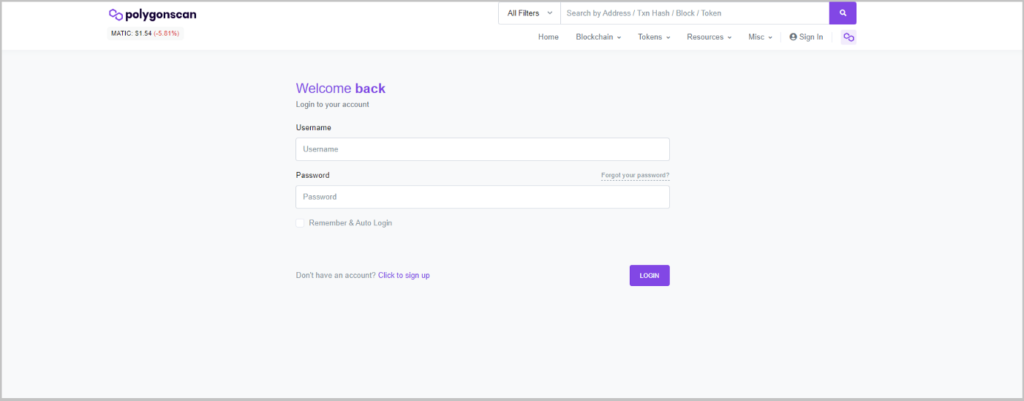
Step 2: Copy your API Key from Polygonscan
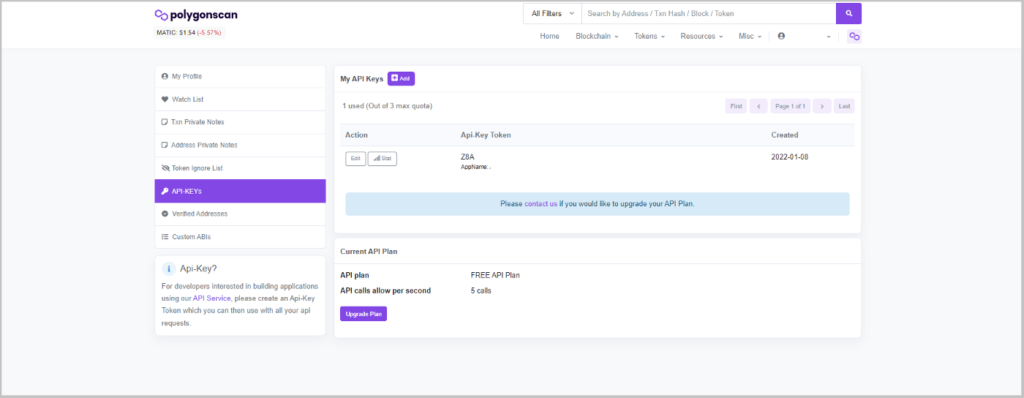
Step 3: Create a .env file
POLYGON_MUMBAI_RPC_PROVIDER = 'RPC Node URL'
PRIVATE_KEY = 'your metamask private key'
POLYGONSCAN_API_KEY = 'your polygonscan api key'
Step 4: Install hardhat-etherscan dependency
hardhat-etherscan is the plugin which allows us to verify the smart contract on Etherscan.
npm install --save-dev @nomiclabs/hardhat-etherscan
or
yarn add --dev @nomiclabs/hardhat-etherscan
Step 5: Update your hardhat.config.js with etherscan
/**
* @type import(‘hardhat/config’).HardhatUserConfig
*/
require(“dotenv”).config();
require(“@nomiclabs/hardhat-ethers”);
require(“@nomiclabs/hardhat-etherscan”);
const { POLYGON_MUMBAI_RPC_PROVIDER, PRIVATE_KEY, POLYGONSCAN_API_KEY } = process.env;
module.exports = {
solidity: “0.8.9”,
defaultNetwork: “mumbai”,
networks: {
hardhat: {},
mumbai: {
url: POLYGON_MUMBAI_RPC_PROVIDER,
accounts: [`0x${PRIVATE_KEY}`], }
}
etherscan: {
apiKey: POLYGONSCAN_API_KEY,
}
};
Step 6: Verify the deployed contract address
npx hardhat verify CONTRACT_ADDR --network mumbai
mumbai is the custom network name that we have used inside the hardhat.config.js.
You will get a response as below:
Successfully verified contract NFT on the block explorer.
https://mumbai.polygonscan.com/address/0x3abae417bbdb0c88cad0998f165e8723f73eb848#code
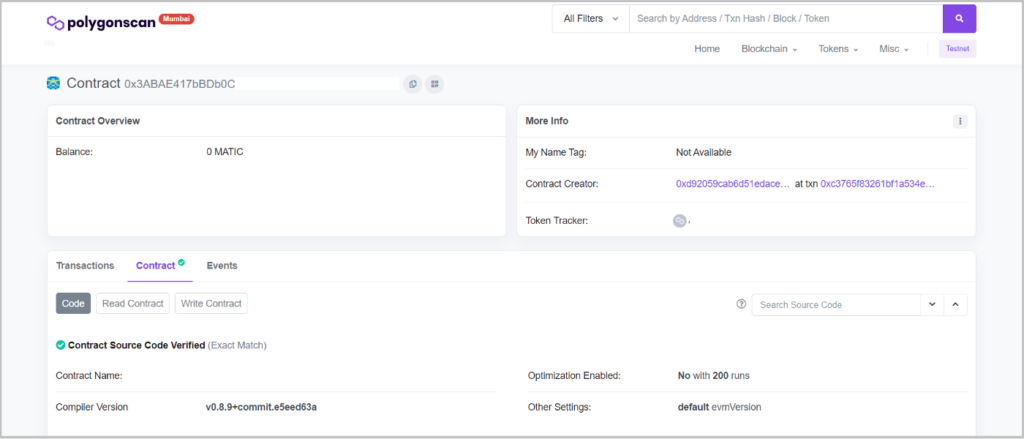
Add on
You can also specify multiple networks with corresponding API Keys to verify on different block explorers.
Update your hardhat.config.js as follows:
/**
* @type import(‘hardhat/config’).HardhatUserConfig
*/
require(“dotenv”).config();
require(“@nomiclabs/hardhat-ethers”);require(“@nomiclabs/hardhat-etherscan”);
const { POLYGON_MUMBAI_RPC_PROVIDER, PRIVATE_KEY, POLYGONSCAN_API_KEY } = process.env;
module.exports = {
solidity: “0.8.9”,
defaultNetwork: “mumbai”,
networks: {
hardhat: {},
mumbai: {
url: POLYGON_MUMBAI_RPC_PROVIDER,
accounts: [`0x${PRIVATE_KEY}`],
}
}
etherscan: {
apiKey: {
//ethereum
mainnet: "ETHERSCAN_API_KEY",
ropsten: "ETHERSCAN_API_KEY",
rinkeby: "ETHERSCAN_API_KEY",
goerli: "ETHERSCAN_API_KEY",
kovan: "ETHERSCAN_API_KEY", //polygon
polygon: "POLYGONSCAN_API_KEY",
polygonMumbai: "POLYGONSCAN_API_KEY"
}
}};